SQLite In Python
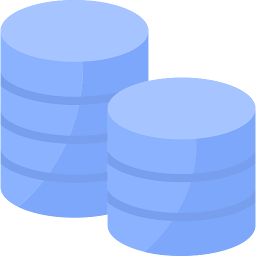
Setup For Work SQLite3 comes with python by default. So, you don't need to install it explicitly. Now create a python script and try with the below code. Connecting Database [ db_connect.py ]: import sqlite3 def create_db(): try: connection = sqlite3.connect('test.db') # if test.db is there then it will connect or it will create a new return connection except(sqlite3.DatabaseError, sqlite3.ProgrammingError): print("DB Connection Error") connection = connect_my_db () Create Table : import sqlite3 import db_connect connection = connect_my_db () cursor_object = connection.cursor() query = ''' CREATE TABLE DemoTable (username text, mail text, phone text, id integer); ''' try: cursor_object.execute(query) print("Table created successfully") except Exception as e: ...